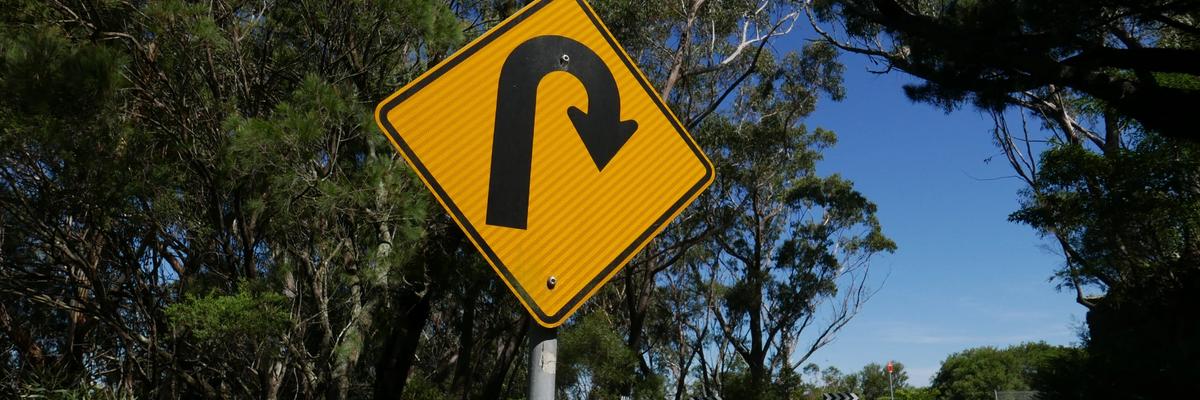
Redirects with Sanity, Next.js and Vercel
September 16, 2021
About a year ago I wrote about how to create redirects with Sanity, Gatsby and Netlify. Since then I've started to use Next.js and Vercel, including for this blog. The switch meant that I simplified my blog post URLs, but to avoid losing some of that SEO juice I'd built up I wanted to make sure any old links pointed to the right place, so I added some redirects with Sanity that are handled automatically by Next.js and Vercel.
Here's how I did it.
Add a redirect document type
Vercel and Next.js expect a different redirect object than Netlify and Gatsby, so the redirect document schema will look slightly different. The fields you need are source
, destination
and permanent
. The last one should be a boolean while the former two should be strings. Your document schema should look like this:
export default {
name: 'redirect',
title: 'Redirect',
type: 'document',
fields: [
{
name: 'source',
title: 'From',
type: 'string',
},
{
name: 'destination',
title: 'To',
type: 'string',
},
{
name: 'permanent',
title: 'Permanent',
type: 'boolean',
initialValue: () => true,
},
],
};
Tip: use the initialValue
magic to set the default value for permanent to true.
Create a script to fetch redirects from Sanity
Create a js file and save it somewhere in your project, you will import this file in your next.config.js
file so it has to be a .js file or else you'll need to transpile it to js before you can use it.
const sanityClient = require('@sanity/client');
const options = {
dataset: 'your-sanity-dataset',
projectId: 'your-sanity-project-id',
token: 'your-sanity-token', // include token if your dataset is private
}
const client = sanityClient(options);
module.exports = async () => {
const query = groq`
*[_type == 'redirect'] {
destination,
source,
permanent
}
`;
const redirects = await client.fetch(query);
return redirects;
}
The above is a pretty simple function that uses a GROQ query to fetch all documents of the type redirect
and queries for the fields destination
, source
and permanent
on those documents.
Update next.config.js
Next, you need to update your next.config.js
file (or create one if you don't have it already) with the following:
const generateRedirects = require('path/to/your/script');
module.exports = {
async redirects() {
return generateRedirects();
},
// other config fields
}
That's it. Next.js/Vercel will now fetch your redirects at build time and handle it for your automatically. It works in development as well, but remember to restart your development server when you make changes to the config.next.js
file as Next won't apply those automatically.
Happy redirecting.