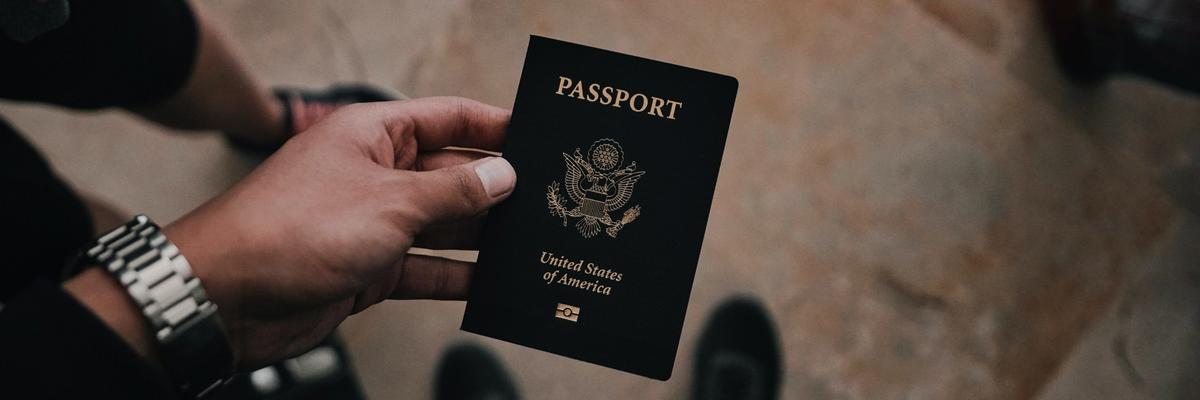
How to add Google Auth with MongoDB Realm
August 27, 2020
When you get started with a Firebase project, it's only a matter of clicking a button to add Google auth to your app. With Realm, there are a few more steps involved. It's no wonder though, seeing as Firebase is a Google service, so it's easy to make a one-click integration.
That said, it's not a hugely complicated task to perform, but it can help to have a simple step by step guide to follow.
Prerequisites
You need to have completed the following prerequisites to follow along with this guide:
- Set up a Realm project
- A Google account
I'm not going to go very deep into implementation details. In my case, I'll implement this in a TypeScript React app, but I'll keep the examples clear of React code. That should mean anyone can use this guide for JavaScript or TypeScript apps with the Realm Web SDK.
Get your Google API client ID
The first step to enable auth with Google is to grab your Google API client ID. I think it's best to let Google tell you how to get your client ID. But if you, like me, find the Google documentation somewhat lacklustre, the gist of it is:
- Go to your Google console.
- Select Credentials from the menu
- Select or create the project you want to add auth to
- If it's a brand new project, click Create credentials and select OAuth Client ID.
- Go through the steps to configure the consent screen; you most likely want to select External when prompted. Don't worry about the ominous-sounding audit. As long as you don't intend to request sensitive user data, it doesn't apply. TIP: if you're fiddling around with this to learn, don't upload a logo as Google will require verification then and you can't delete an already uploaded logo. Trust me, that's what happened to me.
- Once done with the consent screen, go back to credentials and click create credentials again and select OAuth client ID. This time you should be able to go through with the process.
- Copy the client ID when you're through and save it to your app. Also copy the client secret because you will need it in the next step.
Enable Google provider
Head over to your Realm project and navigate to Users->Providers and click Google, then enable the provider and add the client ID and secret.
Add a redirect URI to a page where you will call Realm.handleAuthRedirect();
.
Add a function to handle Google auth and put the following code in it:
async function loginWithGoogle() {
// change SITE_URL to match your site
const redirectUri = `${SITE_URL}/oauth-redirect`;
const credentials = Realm.Credentials.google(redirectUri);
// Calling logIn() opens a Google authentication screen in a new window.
const user = await app.logIn(credentials);
return user;
}
If you piece that together and call that function from your login page, you should get redirected to your OAuth redirect page. However, notice that there's an issue. Realm seems to have forgotten to tell you what callback/redirect-URI you should use. Most likely you'll see an error in the new window/tab that opened, saying something along the lines that the redirect URI doesn't match what you added to the Google project. Just copy that URL and add it to the Google project. Save the changes and try again. It should work like a charm now.
Conclusion
Documentation doesn't always align with reality. When it doesn't, it's useful to document what differs, so you know how to handle it next time you hit the same wall. I've made the mistake of just moving on too many times. This time I wanted to record it for posterity.
If you enjoyed this post about MongoDB Realm, please follow me on Twitter: @BeppeKarlsson to keep up to date on my foray into Realm and other parts of my stack.